ProgressBar Dialog Example In Android
In this tutorial we will learn to implement progress dialog's for spinner progress bar and horizontal progress bar in Android.
We will learn here two types of Progress bar's, Horizontal and Spinner. We use horizontal for downloading and spinner for loading.
Example:
- Create a new project and name it as ProgressBar in Android Studio.
- Design the main layout file as follows.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center_horizontal" android:orientation="vertical" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.hss_24.progressbar.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="20dp" android:text="ANDROID LOVERS" android:textSize="28sp" android:textStyle="bold" /> <Button android:id="@+id/btn_spinner" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="80dp" android:text="Spinner ProgressBar" /> <Button android:id="@+id/btn_horizontal" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Horizontal ProgressBar" /> </LinearLayout>
- Write the code in the main java file as follows.
import android.app.ProgressDialog; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button button_spinner = (Button) findViewById(R.id.btn_spinner); Button button_horizontal = (Button) findViewById(R.id.btn_horizontal); button_spinner.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { ProgressDialog progress_spinner = new ProgressDialog(MainActivity.this); progress_spinner.setMessage("Loading..."); progress_spinner.setProgressStyle(ProgressDialog.STYLE_SPINNER); progress_spinner.show(); } }); button_horizontal.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { ProgressDialog progress_horizontal = new ProgressDialog(MainActivity.this); progress_horizontal.setMessage("Downloading..."); progress_horizontal.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL); progress_horizontal.setIndeterminate(true); progress_horizontal.show(); } }); } }
- Run the Application and enjoy the output.
Download Project
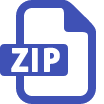
Thank you!!!
Please like and share...
thank you Pavithra M, We are happy to help you
ReplyDelete